Model-View-Presenter Pattern in Java: Enhancing UI Logic Separation for Cleaner Code
Also known as
- MVP
Intent of Model-View-Presenter Design Pattern
MVP aims to separate the user interface (UI) logic from the business logic and model in a software application, enabling easier testing and maintenance.
Detailed Explanation of Model-View-Presenter Pattern with Real-World Examples
Real-world example
Consider a real-world analogy of the Model-View-Presenter (MVP) pattern using a restaurant scenario:
Model: This is the kitchen in a restaurant, where all the cooking and preparation of dishes happens. It's responsible for managing the food ingredients, cooking processes, and ensuring that recipes are followed correctly.
View: This represents the dining area and the menu presented to the customers. It displays the available dishes, takes orders, and shows the final presentation of the food. However, it doesn't decide what's cooked or how it's prepared.
Presenter: Acting as the waiter, the presenter takes the customer's order (input) and communicates it to the kitchen (model). The waiter then brings the prepared food (output) back to the customer in the dining area (view). The waiter ensures that what the customer sees (the menu and food presentation) aligns with what the kitchen can provide, and also updates the view based on the kitchen's capabilities (e.g., out-of-stock items).
In this analogy, the clear separation of roles allows the restaurant to operate efficiently: the kitchen focuses on food preparation, the dining area on customer interaction, and the waiter bridges the two, ensuring smooth operation and customer satisfaction.
In plain words
The Model-View-Presenter (MVP) pattern separates the user interface, business logic, and data interaction in an application, with the presenter mediating between the view and the model to facilitate clear communication and updates. Java developers use MVP to improve application structure.
Wikipedia says
Model–view–presenter (MVP) is a derivation of the model–view–controller (MVC) architectural pattern, and is used mostly for building user interfaces. In MVP, the presenter assumes the functionality of the "middle-man". In MVP, all presentation logic is pushed to the presenter.
Architecture diagram
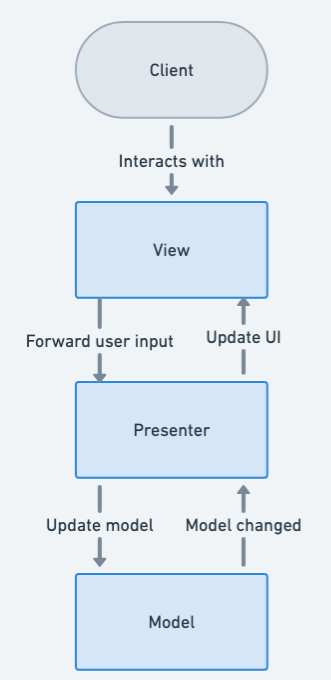
Programmatic Example of Model-View-Presenter Pattern in Java
The Model-View-Presenter (MVP) design pattern is a derivative of the well-known Model-View-Controller (MVC) pattern. It aims to separate the application's logic (Model), GUIs (View), and the way that the user's actions update the application's logic (Presenter). This separation of concerns makes the application easier to manage, extend, and test.
Let's break down the MVP pattern using the provided code:
- Model: The Model represents the application's logic. In our case, the
FileLoader
class is the Model. It's responsible for handling the file loading process.
@Getter
public class FileLoader implements Serializable {
//...
public void setFileName(String fileName) {
this.fileName = fileName;
}
public boolean fileExists() {
//...
}
public String loadData() {
//...
}
}
- View: The View is responsible for displaying the data provided by the Model. Here, the
FileSelectorView
interface and its implementationFileSelectorJFrame
represent the View. They define how to display data and messages to the user.
public interface FileSelectorView {
//...
void setPresenter(FileSelectorPresenter presenter);
void open();
void close();
void showMessage(String message);
void displayData(String data);
String getFileName();
}
public class FileSelectorJFrame implements FileSelectorView {
//...
@Override
public void displayData(String data) {
this.dataDisplayed = true;
}
}
- Presenter: The Presenter acts as a bridge between the Model and the View. It reacts to the user's actions and updates the View accordingly. In our example, the
FileSelectorPresenter
class is the Presenter.
public class FileSelectorPresenter implements Serializable {
//...
public void setLoader(FileLoader loader) {
this.loader = loader;
}
public void start() {
view.setPresenter(this);
view.open();
}
public void fileNameChanged() {
loader.setFileName(view.getFileName());
}
public void confirmed() {
//...
}
public void cancelled() {
view.close();
}
}
Finally, we wire up the Presenter, the View, and the Model in the App
class:
public class App {
public static void main(String[] args) {
var loader = new FileLoader();
var frame = new FileSelectorJFrame();
var presenter = new FileSelectorPresenter(frame);
presenter.setLoader(loader);
presenter.start();
}
}
In this setup, the App
class creates instances of the Model, View, and Presenter. It then connects these instances, forming the MVP triad. The Presenter is given a reference to the View, and the Model is set on the Presenter. Finally, the Presenter is started, which in turn opens the View.
When to Use the Model-View-Presenter Pattern in Java
Use MVP in applications where a clear separation of concerns is needed between the presentation layer and the underlying business logic. It's particularly useful in client-server applications and enterprise-level applications.
Real-World Applications of Model-View-Presenter Pattern in Java
- Desktop applications like those built using Java Swing or JavaFX.
- Web applications with complex user interfaces and business logic.
Benefits and Trade-offs of Model-View-Presenter Pattern
Benefits:
- Enhances testability of UI logic by allowing the presenter to be tested separately from the view.
- Promotes a clean separation of concerns, making the application easier to manage and extend.
- Facilitates easier UI updates without affecting the business logic.
Trade-offs:
- Increases complexity with more classes and interfaces.
- Requires careful design to avoid over-coupling between the presenter and the view.
Related Java Design Patterns
- Model-View-Controller (MVC): MVP is often considered a variant of MVC where the presenter takes over the controller's role in managing user input and updating the model.
- Model-View-ViewModel (MVVM): Similar to MVP but adapted for frameworks like WPF or frameworks that support data binding, making the view update automatically when the model changes.