Component Pattern in Java: Simplifying Complex Systems with Reusable Components
Also known as
- Entity-Component-System (ECS)
- Component-Entity-System (CES)
- Component-Based Architecture (CBA)
Intent of Component Design Pattern
The Component design pattern organizes code into reusable, interchangeable components, promoting flexibility, modularity, and ease of maintenance. This pattern is especially useful in game development, enabling entities to be configured with diverse behaviors dynamically.
Detailed Explanation of Component Pattern with Real-World Examples
Real-world example
Consider a video game with a graphics component and a sound component. Including both in a single Java class can create maintenance challenges due to lengthy code and potential conflicts from different teams working on the same class. The Component design pattern resolves this by creating individual component classes for graphics and sound, allowing flexible and independent development. This modular approach enhances maintainability and scalability.
In plain words
The component design pattern provides a single attribute to be accessible by numerous objects without requiring the existence of a relationship between the objects themselves.
Architecture diagram
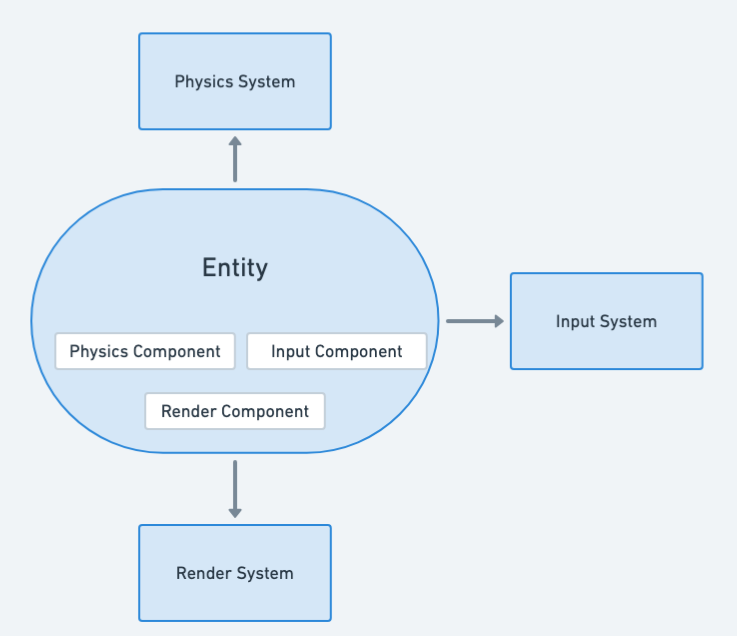
Programmatic Example of Component Pattern in Java
The App
class creates a demonstration of the use of the component pattern by creating two different objects which inherit a small collection of individual components that are modifiable.
public final class App {
public static void main(String[] args) {
final var player = GameObject.createPlayer();
final var npc = GameObject.createNpc();
LOGGER.info("Player Update:");
player.update(KeyEvent.KEY_LOCATION_LEFT);
LOGGER.info("NPC Update:");
npc.demoUpdate();
}
}
Much of the program exists within the GameObject
class, within this class, the player and NPC object create methods are set up. Additionally, this class also consists of the method calls used to update/alter information of the object's components.
public class GameObject {
private final InputComponent inputComponent;
private final PhysicComponent physicComponent;
private final GraphicComponent graphicComponent;
public String name;
public int velocity = 0;
public int coordinate = 0;
public static GameObject createPlayer() {
return new GameObject(new PlayerInputComponent(),
new ObjectPhysicComponent(),
new ObjectGraphicComponent(),
"player");
}
public static GameObject createNpc() {
return new GameObject(
new DemoInputComponent(),
new ObjectPhysicComponent(),
new ObjectGraphicComponent(),
"npc");
}
public void demoUpdate() {
inputComponent.update(this);
physicComponent.update(this);
graphicComponent.update(this);
}
public void update(int e) {
inputComponent.update(this, e);
physicComponent.update(this);
graphicComponent.update(this);
}
public void updateVelocity(int acceleration) {
this.velocity += acceleration;
}
public void updateCoordinate() {
this.coordinate += this.velocity;
}
}
Upon opening the component package, the collection of components are revealed. These components provide the interface for objects to inherit these domains. The PlayerInputComponent
class shown below updates the object's velocity characteristic based on user's key event input.
public class PlayerInputComponent implements InputComponent {
private static final int walkAcceleration = 1;
@Override
public void update(GameObject gameObject, int e) {
switch (e) {
case KeyEvent.KEY_LOCATION_LEFT -> {
gameObject.updateVelocity(-WALK_ACCELERATION);
LOGGER.info(gameObject.getName() + " has moved left.");
}
case KeyEvent.KEY_LOCATION_RIGHT -> {
gameObject.updateVelocity(WALK_ACCELERATION);
LOGGER.info(gameObject.getName() + " has moved right.");
}
default -> {
LOGGER.info(gameObject.getName() + "'s velocity is unchanged due to the invalid input");
gameObject.updateVelocity(0);
} // incorrect input
}
}
}
When to Use the Component Pattern in Java
- Used in game development and simulations where game entities (e.g., characters, items) can have a dynamic set of abilities or states.
- Suitable for systems requiring high modularity and systems where entities might need to change behavior at runtime without inheritance hierarchies.
Real-World Applications of Component Pattern in Java
The Component pattern is ideal for game development and simulations where entities like characters and items have dynamic abilities or states. It suits systems requiring high modularity and scenarios where entities need to change behavior at runtime without relying on inheritance hierarchies, enhancing flexibility and maintainability.
Benefits and Trade-offs of Component Pattern
Benefits:
- Flexibility and Reusability: Components can be reused across different entities, making it easier to add new features or modify existing ones.
- Decoupling: Reduces dependencies between game entity states and behaviors, facilitating easier changes and maintenance.
- Dynamic Composition: Entities can alter their behavior at runtime by adding or removing components, providing significant flexibility in game design.
Trade-offs:
- Complexity: Can introduce additional complexity in system architecture, particularly in managing dependencies and communications between components.
- Performance Considerations: Depending on implementation, may incur a performance overhead due to indirection and dynamic behavior, especially critical in high-performance game loops.
Related Java Design Patterns
- Decorator: Similar concept of adding responsibilities dynamically, but without the focus on game entities.
- Flyweight: Can be used in conjunction with the Component pattern to share component instances among many entities to save memory.
- Observer: Often used in Component systems to communicate state changes between components.